CouchDB connection
1. Log into Apiqcloud
2. Create an environment with CouchDB database:
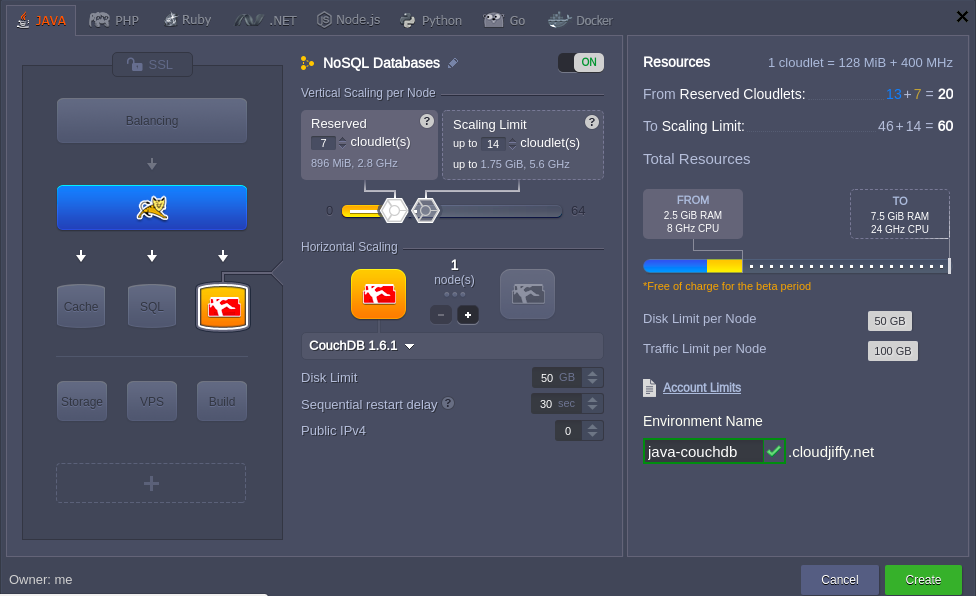
3. Check your email box for getting credentials to access CouchDB node:


4. Then create a file mydb.cfg in Config Manager (folder HOME). This file contains information on connecting to CouchDB. This info will be read by your application:
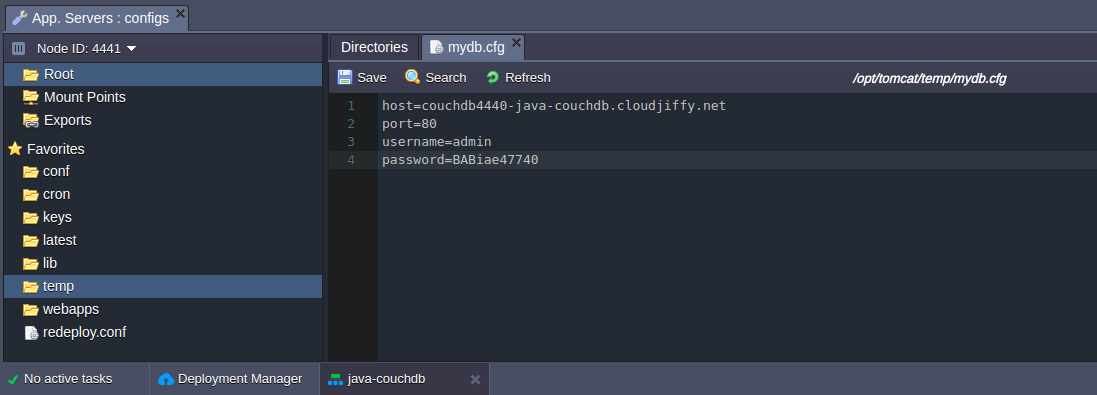
5. In this step, you have to prepare part of java code that creates a connection to your DB
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
import com.fourspaces.couchdb.*; import java.io.FileInputStream; import java.io.IOException; import java.util.Properties; import java.util.logging.Level; import java.util.logging.Logger; public class CouchdbConnector { public boolean createDatabase(String name) { try { //Read file mydb.cfg Properties prop = new Properties(); prop.load( new FileInputStream(System.getProperty( "user.home" ) + "/mydb.cfg" )); //Getting info we need: host, port, username, password String host = prop.getProperty( "host" ).toString(); int port = Integer.parseInt(prop.getProperty( "port" ).toString()); String username = prop.getProperty( "username" ).toString(); String password = prop.getProperty( "password" ).toString(); Session dbSession = new Session(host, port, username, password); dbSession.createDatabase(name); try { dbSession.getDatabase(name); } catch (Exception e) { return false ; } } catch (IOException ex) { Logger.getLogger(CouchdbConnector. class .getName()).log(Level.SEVERE, null , ex); } return true ; } } |
NOTE: you have to upload all libraries that CouchDB connection needs!
6. Deploy application: CouchDB.war
7. Run deployed application and input database name you want to create:

Input database name:
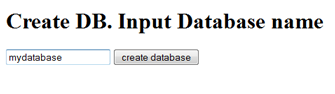
8. Check CouchDB server if it contains a "new" database:
- run CouchDB instance in a browser;
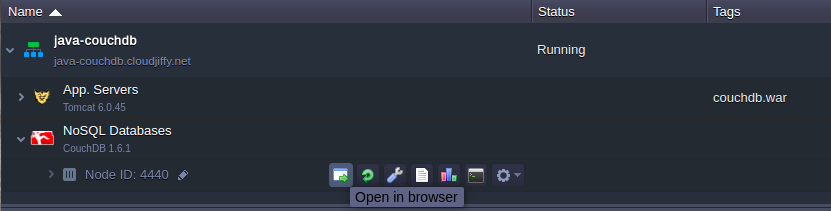
- input credentials that you got on e-mail;

- check database presence.

