Connection to PostgreSQL for PHP Applications
Create Environment
1. Log into the Apiqcloud.
2. Create PHP environment with PostgreSQL database: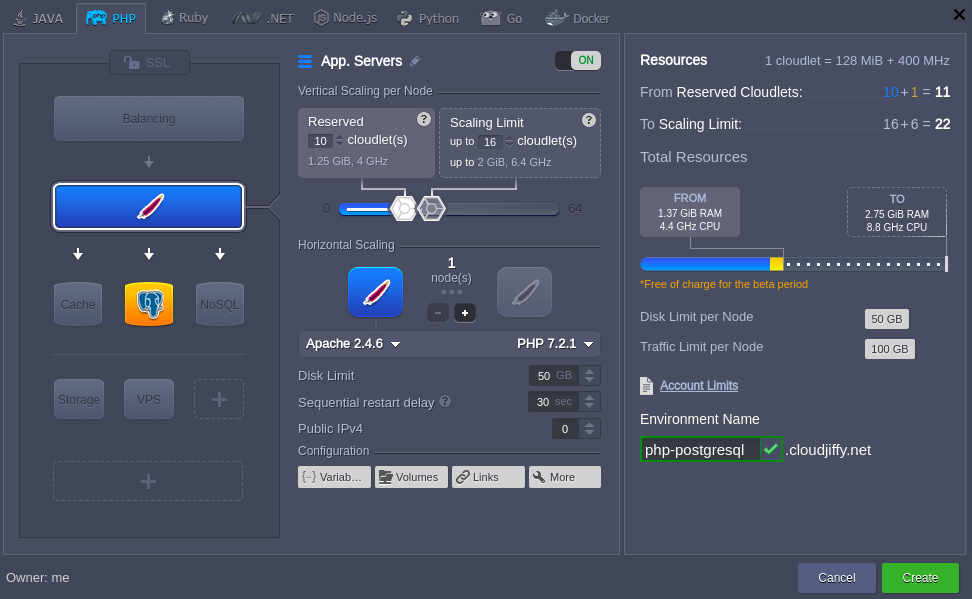
3. Check your e-mail - your inbox should have a message with database login and password:
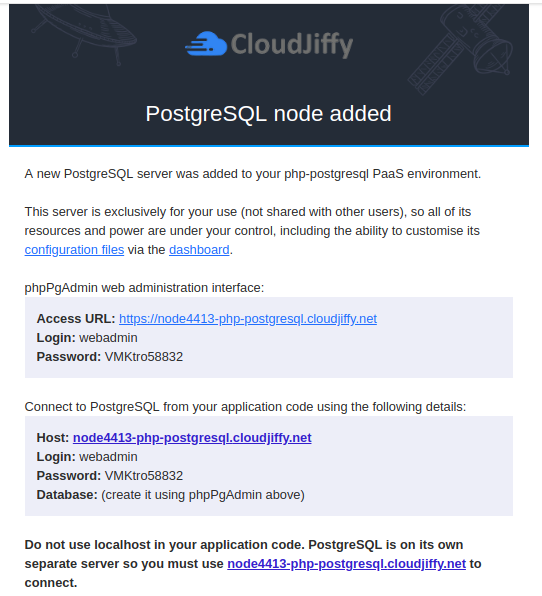
Now, you can upload your PHP application package and deploy it to the environment.
Database Connection
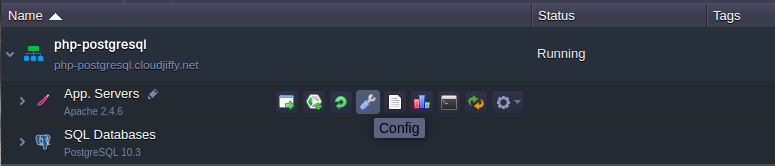
2. Navigate to etc folder and open php.ini file.
3. Add extension=pgsql.so line after extension=mysql.so, like it is shown in the image below: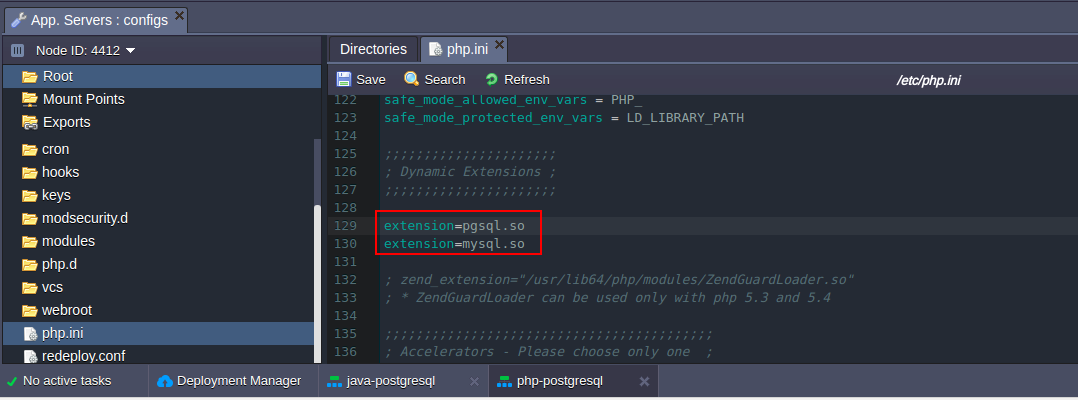
4. Save the changes and Restart nodes for Apache server.
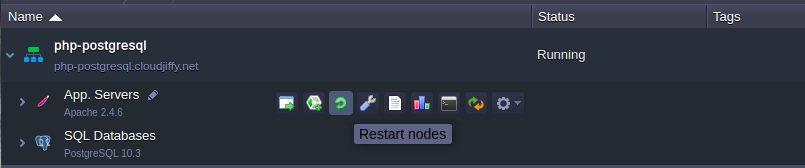
5. There are 2 main PG functions for connection:
- Connection to your postgresql database:
- host - Postgres server address that you received by email (note that it must be without http://):
postgres{node_id}-{your_env_name}.{hoster_domain}
- port - by default 5432
- dbname - name of your database
- user - by default is "webadmin"
- password - the password that you received via email
-
Closing connection to postgresql database: pg_close()
All Postgres functions you can see here.
6. You need to write necessary functions in every *.php page, which should be connected to the database.Connection Check Up
- check the connection using this code:
1
2
3
4
5
6
7
8
9
10
11
|
<?php $dbconn = pg_connect( "host=postgres-demo.jelastic.com port=5432 dbname=postgres-demo user=webadmin password=password" ); //connect to a database named "postgres" on the host "host" with a username and password if (! $dbconn ){ echo "<center><h1>Doesn't work =(</h1></center>" ; } else echo "<center><h1>Good connection</h1></center>" ; pg_close( $dbconn ); ?> |
- execute simple request and output it into table:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
<?php $conn = pg_connect( "host=postgres-demo.jelastic.com port=5432 dbname=postgres-demo user=webadmin password=password" ); if (! $conn ) { echo "An error occured.\n" ; exit ; } $result = pg_query( $conn , "SELECT * FROM test_table" ); if (! $result ) { echo "An error occured.\n" ; exit ; } while ( $row = pg_fetch_row( $result )) { echo "value1: $row[0] value2: $row[1]" ; echo "<br />\n" ; } ?> |
Now, you can use connection to PostgreSQL database for your PHP application.